I have a video library developed using C# MVC5. I import videos into my video library and try to display "stream" them when a user clicks on the video in the front end.
The video is being rendered using the HTML5 video tag, but according to what I have read, there needs to be separate video files for each of the different browsers.
What is the best way to achieve this? The videos being imported are in MP4 format, which plays in Chrome but not in the other browsers. I see some articles on using ffmpeg to create "versions" of the video.
Any advice?
Related
I'm creating a tool to scrape and search the Amazon music database, it then cross references with google to display a bunch of information about the songs. One thing I want to add if possible, is to play the sound from the form using the media link scraped form amazons webpage.
This is the media link:
https://d2q1srilgjznst.cloudfront.net/64%2F30%2F232646106_S64.mp3?response-content-type=audio%2Fmpeg&Expires=1534727161&Signature=HF~qj5XP2WRKBJlT0VT1hDIq4cieH7SWcgnxRUGWm045iqIV4L7YTxlLihQdde9ZNrL97xf8zkfatRn-YxGYh0Yk1RmCHHI6H53j1wjig36KsHPdlnwoEp7qM45NsNhYf4IfXXjHzQ-tnkDfocpFSHMqss9FosYXWCf9qGrWKTo_&Key-Pair-Id=APKAJVZTZLZ7I5XDXGUQ
I have already scraped the data needed, I just am unsure how I can go about playing this on my C# application?
I don't have any code to show as searching on google I couldn't find a single thing relevant to this, or if its even possible. The closest I found was this, for playing media files.
System.Media.SoundPlayer player = new System.Media.SoundPlayer(#"");
player.Play();
The other question is for MP3 files, this is for data sourced online.
I have a problem regarding unity 3d video stream, I want to open I can open mp4 videos but with the video player I can't open m3u8 video streams, is there a way to open this kind of videos.Is there any way to play it via u3dxt plugin
EasyMovieTexture is able to play m3u8 format
The AVPro Video plugin for Unity has really good adaptive video file support (both DASH and HLS/m3u8) and supports many platforms. Much easier to use compared to EasyMovieTexture as well.
Using AVPro Video and the Vimeo Unity plugin together is also really helpful if you need to host and transcode videos to the m3u8 format.
I'm writing a web app in .NET MVC4 and would like to use Markdown. I understand there are a few open source C# Markdown libraries, but I haven't found one that obviously supports embedding youtube or Vimeo videos inside Markdown text.
Does anyone know if it's possible?
The Solution using Standard Markdown ( not iFrame! )
Using an iframe is not the "obvious" solution... especially if the Markdown parser (or publishing platform) you are using does not support inlining content from a different website ... Instead you can "fake it" by including a valid linked-image in your Markdown file, using this format:
[](http://www.youtube.com/watch?v=YOUTUBE_VIDEO_ID_HERE "Video Title")
Explanation of the Markdown
If this markdown snippet looks complicated, break it down into two parts:
an image

wrapped in a link
[link text](http//example.io/my-link "link title")
Example using Valid Markdown and YouTube Thumbnail:
We are sourcing the thumbnail image directly from YouTube and linking to the actual video, so when the person clicks the image/thumbnail they will be taken to the video.
Code:
[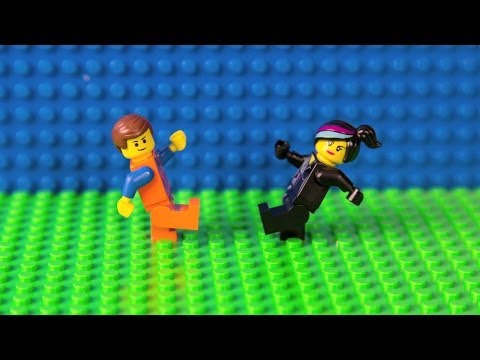](https://www.youtube.com/watch?v=StTqXEQ2l-Y "Everything Is AWESOME")
OR If you want to give readers a visual cue that the image/thumbnail is actually a playable video, take your own screenshot of the video in YouTube and use that as the thumbnail instead.
Example using Screenshot with Video Controls as Visual Cue:
Code:
[](https://youtu.be/StTqXEQ2l-Y?t=35s "Everything Is AWESOME")
Clear Advantages
While this requires a couple of extra steps (a) taking the screenshot of the video and (b) uploading it so you can use the image as your thumbnail it does have 3 clear advantages:
The person reading your markdown (or resulting html page) has a visual cue telling them they can watch the video (video controls encourage clicking)
You can chose a specific frame in the video to use as the thumbnail (thus making your content more engaging)
You can link to a specific time in the video from which play will start when the linked-image is clicked. (in our case from 35 seconds)
Taking a screenshot takes a few seconds and there are keyboard shortcuts for each OS which copy the screenshot to your clipboard which means you can paste it for even faster upload.
Not Only C#
And since this is 100% Standard markdown, it works everywhere (not just for the C# parser!) ... try it on GitHub, Redit or Ghost!
Vimeo
This approach also works with Vimeo videos
Example
Code
[](https://vimeo.com/3514904 "Little red riding hood - Click to Watch!")
Notes:
How to take screenshot: http://www.take-a-screenshot.org/ (all platforms!)
Upload Thumbnail Image: Once you've taken your screenshot you can drag-and-drop it into imgur.com to upload and immediately use it as your thumbnail; couldn't be easier!
YouTube thumbnail info: How do I get a YouTube video thumbnail from the YouTube API?
Markdown format borrowed/modified/expanded from: Embed a YouTube video
You can use inline HTML to embed your video.
# this is a *markdown* document
<iframe title="YouTube video player" width="480" height="390" src="http://www.youtube.com/watch?v=TheVideoID?autoplay=1" frameborder="0" allowfullscreen></iframe>
with a **youtube** video embedded
<iframe width="560" height="315" src="https://www.youtube.com/embed/-mUJnKI3ipI" frameborder="0" allowfullscreen></iframe>
Markdown won't let you embed videos, Basically the answers posted here are explaining to have a image link, which is clearly not what an embed means. So the answer to whether you can embed a video or not is "NO You can't".
You should be able to use the HTML5 <video> element. Someone tell me if this doesn't work.
(Just spotted this, many years late :-) , as I want to add video support to my md2pptx Markdown to PowerPoint open source tool.)
What about the syntax for embedding image, applied to other media?

Oembed is interesting to make embedding easier: users just have to paste the URL instead of an iframe code.
For video, it could be

How to download a video file from YouTube as .FLV or .MP4 format to hard disk using .NET?
I want to create a small YouTube downloader application which asks the user for the specefic link, when i click the download button, the download process start downloading the video as an FLV file directly from YouTube server to the hard disk.
I'm not asking here for the detailed procedure but i want to know only how to start ? is there a YouTube c# api which handle this process.
How to download an Flv video file from YouTube to hard disk using C# ?
Thank you !
I think this project is interesting
https://github.com/flagbug/YoutubeExtractor
try run an embed browser and add event handler to grep URLs of all resources on webpage, then you can study the protocol used by youtube.
changing the HTML request header to simulate an iOS device may force youtube use HTML5 player , instead of flash player ( if the above method cannot track network activities inside a flash player )
Find an open source program that can do this, and read the portion of the source code that forms the youtube requests.
For example, gPodder is my favorite application of this type, and would be a good choice if you read python code. But I'm sure you can find others.
Once you find out the right format for requests, you can use C#'s HTTP classes such as HttpWebRequest. Finally, save the data to a file.
Google has also provided some documentation for accessing YouTube from .NET, but this is more related to the social networking aspects of the site than the video content.
It is one thing to stream a video to a html page and render the web page using a .NET Form and .NET Web Browser. My Question to you is whether it is possible to render or stream video to a video player rather than an embedded video in a html page. Is that called breaking in ?
Have you checked out MSDN and the DirectX api Video object?